When looking for a great themed slot, do not miss Monty Python’s Spamalot, with 5 reels, 20 paylines, by Playtech. The musical comedy, also based on a film from 1976, about Monty Python and the Holy Grail, have done a lot for people, entertaining them, and now it is the turn of the Playtech Video Slot themed on Monty.
In Python every class can have instance attributes. By default Pythonuses a dict to store an object’s instance attributes. This is reallyhelpful as it allows setting arbitrary new attributes at runtime.
- Slot Machine in Python Encapsulate functionality in a class. I'd create a class called SlotMachine that holds the global state. It should store. Keep related constants in an Enum. This also helps avoid.
- Simple, expandable, customizable slot machine. Help the Python Software Foundation raise $60,000 USD by December 31st! Building the PSF Q4 Fundraiser.
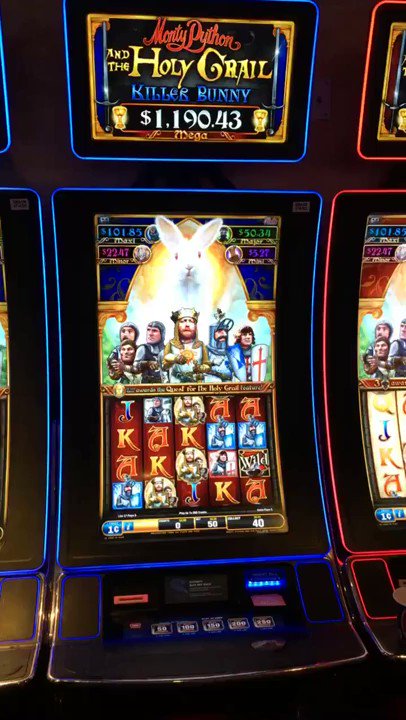
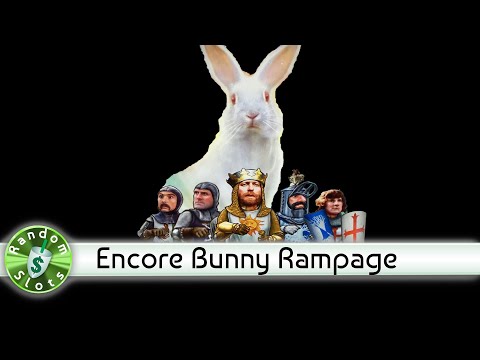
However, for small classes with known attributes it might be abottleneck. The dict
wastes a lot of RAM. Python can’t just allocatea static amount of memory at object creation to store all theattributes. Therefore it sucks a lot of RAM if you create a lot ofobjects (I am talking in thousands and millions). Still there is a wayto circumvent this issue. It involves the usage of __slots__
totell Python not to use a dict, and only allocate space for a fixed setof attributes. Here is an example with and without __slots__
:
Without__slots__
:
Monty Python Slot Machine
With__slots__
:
The second piece of code will reduce the burden on your RAM. Some peoplehave seen almost 40 to 50% reduction in RAM usage by using thistechnique.
On a sidenote, you might want to give PyPy a try. It does all of theseoptimizations by default.
Below you can see an example showing exact memory usage with and without __slots__
done in IPython thanks to https://github.com/ianozsvald/ipython_memory_usage
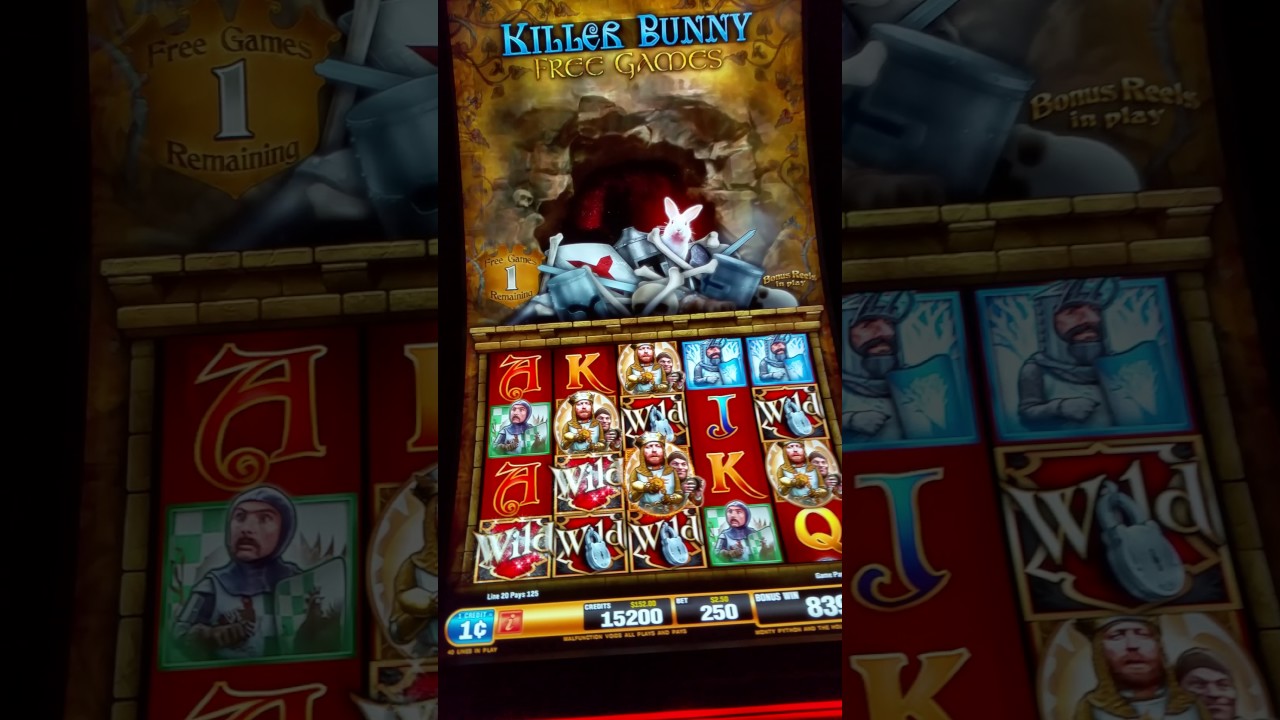
Monty Python Slot Machine
- #use python to write a code that allows the user to play a simulated slot machine using tokens
- #give them 100 tokens to start out with but every game makes them loose one
- # if they spin 3 of the same number they win tokens 1 = 4, 2 = 8, 3 = 12
- tokens =100
- print'Welcome to slots! You start with 100 coins and loose one each time you spin the slot machine'
- print'If you spin 3 of the same number you win coins'
- yes_no =raw_input('would yo like to play slots? Y or N')
- tokens = tokens - 1
- number2 =random.randint(1,3)
- print number1 ,',', number2 ,',', number3
- if number1 1:
- if number1 2:
- if number1 3:
- print'you have', tokens ,'tokens'
- yes_no =raw_input('would yo like to play slots? Y or N')
- print'goodbye'
- print'you ran out of tokens:
- print 'invalid input'